components
The components directory contains your Vue.js components. Components are what makes up the different parts of your page and can be reused and imported into your pages, layouts and even other components.
Fetching Data
To access asynchronous data from an API in your components you can use Nuxt fetch()
hook.
Using $fetchState.pending
we can show a message when the data is waiting to be loaded and using $fetchState.error
we can show an error message if there is an error fetching the data. When using fetch we must declare the data in the data property. This then gets filled with the data that comes from the fetch.
<template>
<div>
<p v-if="$fetchState.pending">Loading....</p>
<p v-else-if="$fetchState.error">Error while fetching mountains</p>
<ul v-else>
<li v-for="(mountain, index) in mountains" :key="index">
{{ mountain.title }}
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
mountains: []
}
},
async fetch() {
this.mountains = await fetch(
'https://api.nuxtjs.dev/mountains'
).then(res => res.json())
}
}
</script>
See the chapter on fetch() for more details on how fetch works
Components Discovery
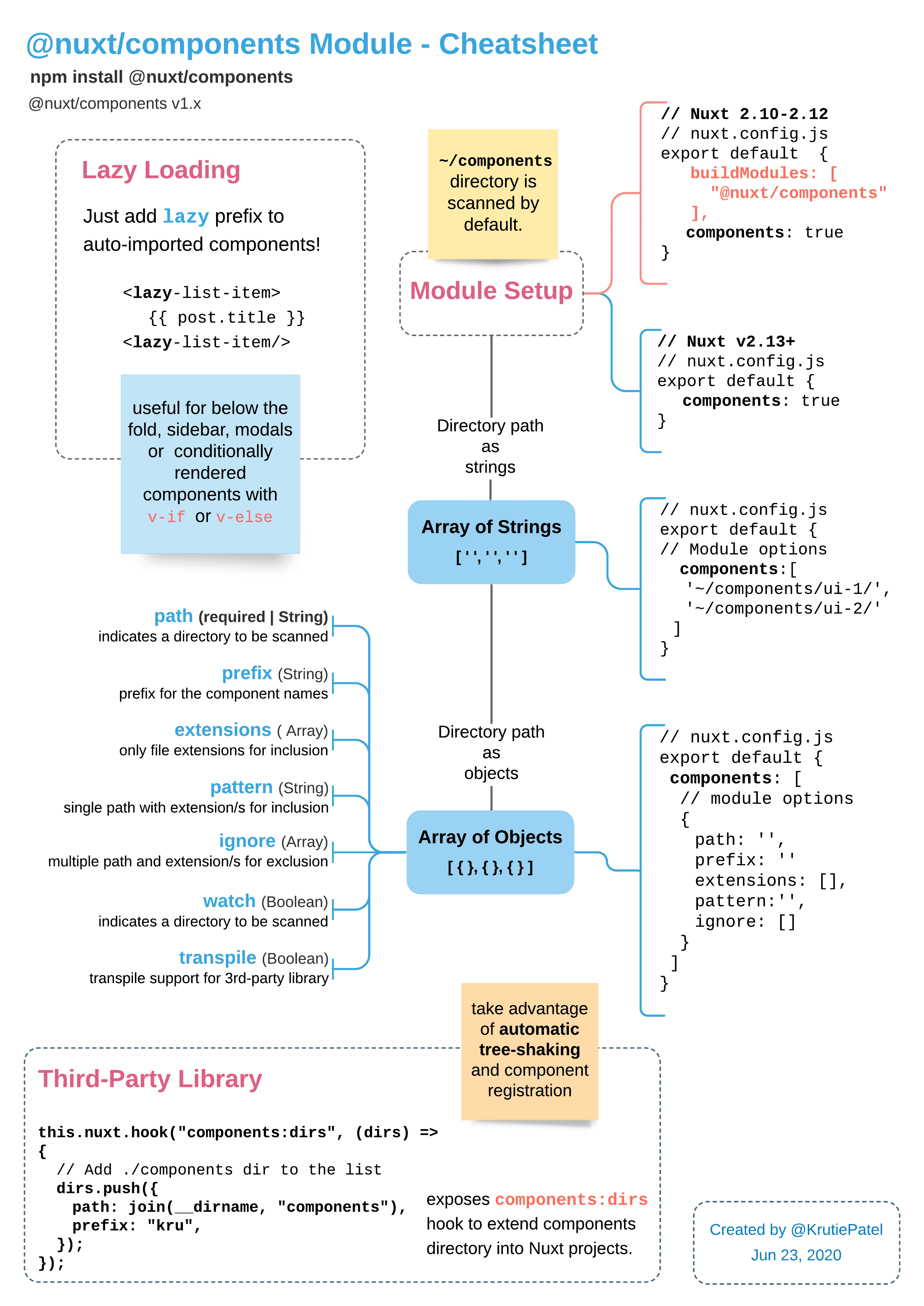
Starting from v2.13
, Nuxt can auto import your components when used in your templates, to activate this feature, set components: true
in your configuration:
export default {
components: true
}
Once you create your components in the components directory they will then be available to be auto imported.
components/
TheHeader.vue
TheFooter.vue
<template>
<div>
<TheHeader />
<Nuxt />
<TheFooter />
</div>
</template>
Dynamic Imports
To dynamically import a component also known, as lazy loading a component, all you need to do is add the Lazy
prefix in your templates.
<template>
<div>
<TheHeader />
<Nuxt />
<LazyTheFooter />
</div>
</template>
Using the lazy prefix you can also dynamically import a component when an event is triggered.
<template>
<div>
<h1>Mountains</h1>
<LazyMountainsList v-if="show" />
<button v-if="!show" @click="showList">Show List</button>
</div>
</template>
<script>
export default {
data() {
return {
show: false
}
},
methods: {
showList() {
this.show = true
}
}
}
</script>
Nested Directories
If you have components in nested directories such as:
components/
base/
Button.vue
The component name will be based on its own filename. Therefore the component will be:
<button />
We recommend you use the directory name in the filename for clarity
components/
base/
BaseButton.vue
However if you want to keep the filename as Button.vue then you can use the prefix option in the nuxt config to add a prefix to all components in a specific folder.
components/
base/
Button.vue
components: {
dirs: [
'~/components',
{
path: '~/components/base/',
prefix: 'Base'
}
]
}
And now in your template you can use the BaseButton instead of Button without having to make changes to the name of your Button.vue
file.
<BaseButton />
To learn more about the components module